Whether you're localizing an application, translating datasets, or preparing content for international audiences, the process can be challenging if you're not familiar with the steps involved.
Luckily, the Lingvanex On-premise Machine Translation Software provides a simple solution for translating JSON content. Although the software doesn't have a specific JSON localization feature, we can achieve the desired result by translating the individual elements within the JSON structure.
In this guide, we'll walk you through the process of translating JSON files using the Lingvanex Translation Software. By following these steps, you'll be able to tackle any JSON language translation project with ease.
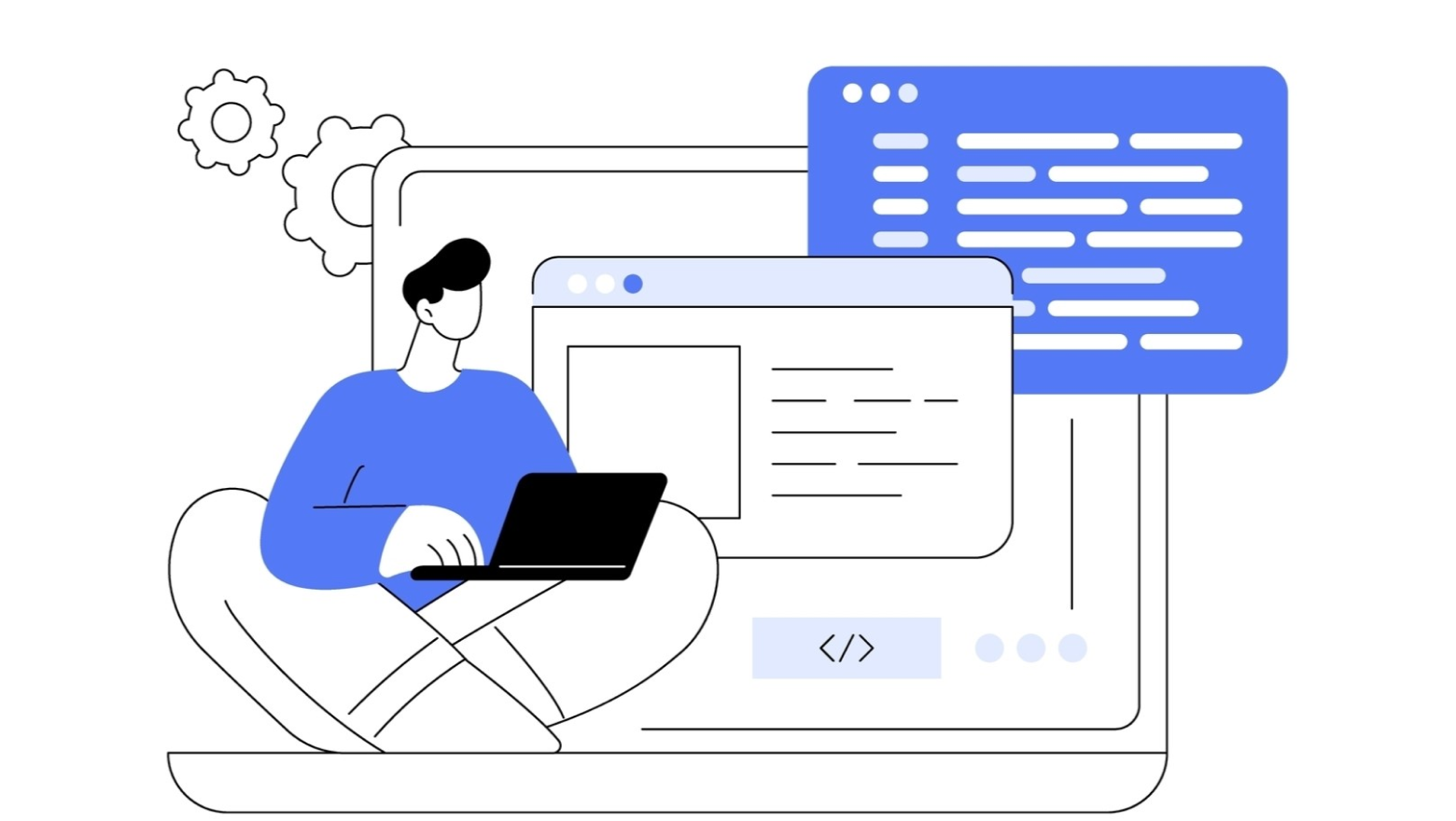
Understanding the Translation Process
The translation of a JSON file involves several key steps:
1. Reading the JSON file;
2. Parsing the JSON into a structure your programming language can work with;
3. Recursively going through this structure, translating text elements;
4. Rebuilding the JSON with translated content;
5. Outputting the translated JSON.
Preparing Your JSON File
Ensure your JSON file is well-formed and free of syntax errors. It's advisable to validate your file using a JSON linter before attempting translation. Remember the file location as you'll need to specify this path in the script.
Choosing Your Programming Environment
This guide provides examples in three popular programming languages: Python, JavaScript (for Node.js), and Bash. The JavaScript examples can be run in both Node.js and web browsers (though browser-based execution won't support functionalities like fs.readFile which require server-side environments). Each language has its strengths, so choose the one that aligns best with your skills and project requirements.
Python offers excellent library support for JSON data translation, JavaScript is great for web-based projects, and Bash is ideal for quick command-line operations.
Using the Lingvanex translation API, you can easily implement machine translation for JSON in your chosen programming environment.
Python Implementation
This Python script does the following:
1. These lines import modules to work with JSON, check collection types, and send HTTP requests. In this example, we use the requests package to interact with the translation server. If it's not already installed in your system, run pip install requests before executing the script.
import json
from collections.abc import Mapping, Sequence
import requests
2. Here, we set the URL of the private translation server, the source language, the target language, and the path to the JSON file.
_PRIVATE_TRANSLATOR_URL: str = 'https://your-server-translator-url/api/translate' # Translation server URL
_SOURCE_LANGUAGE: str = 'en' # Source language
_TARGET_LANGUAGE: str = 'fr' # Target language
_JSON_FILEPATH: str = '/path-to-your-json-file' # Path to the JSON file
3. This function sends a request to the translation server with the text to be translated and returns the translated text. If the server returns an error, it will be handled.
def _translate(text: str) -> str:
'''Translate text via private translator server'''
request_body = {
'source': _SOURCE_LANGUAGE,
'target': _TARGET_LANGUAGE,
'q': text,
}
with requests.post(url=_PRIVATE_TRANSLATOR_URL, data=request_body) as response:
response.raise_for_status() # In case of an error on the translator API side
return response.json()['translatedText']
4. This function recursively translates all strings in the JSON object. If the value is a string, the function translates it. If it's a dictionary or list, the function processes them accordingly.
def _translate_json_document(value: object) -> object:
'''
Recursively translates the object passed to this function.
If the passed object is Mapping - translates only the values.
:param value: One of the possible types that a JSON object can contain.
'''
if isinstance(value, str):
return _translate(value)
# Check if value is a List or a Dictionary and handle it appropriately
if isinstance(value, Mapping):
new_values = {}
iterable_values = value.items()
elif isinstance(value, Sequence):
new_values = [None]*len(value)
iterable_values = enumerate(value)
else:
return value
for key, item in iterable_values:
new_values[key] = _translate_json_document(item)
return new_values
5. This function reads the JSON file, parses and translates it using the previously defined functions, and prints the translated JSON to the console. The comments at the end of the function show how to save the translated JSON to a file if needed.
def _main() -> None:
with open(_JSON_FILEPATH, 'r') as json_file:
data = json.load(json_file) # Convert JSON to Python object
translated = _translate_json_document(data)
translated_json = json.dumps(translated, indent=4, ensure_ascii=False)
print(translated_json)
# Uncomment code below in case if you need to write translated JSON to file
# target_translated_json_filepath: str = f'{_JSON_FILEPATH}.output.json' # Replace with your filepath
# with open(target_translated_json_filepath, 'w') as file:
# file.write(translated_json)
6. This line runs the main function if the script is executed as the main module, rather than being imported by another module.
if __name__ == '__main__':
_main()
JavaScript (Node.js) Implementation
If you prefer JavaScript, here's how you can do it with Node.js:
1. This line imports the fs (file system) module to work with files. We use promises for asynchronous operations.
const fs = require('fs').promises;
2. Here, we set the source language, target language, URL of the private translation server, and the path to the JSON file.
const SOURCE_LANGUAGE = 'en'; // Source language
const TARGET_LANGUAGE = 'fr'; // Target language
const PRIVATE_TRANSLATOR_URL = 'https://your-server-translator-url/api/translate'; // Translation server URL
const JSON_FILE_PATH = '/your-json-file-path'; // Path to the JSON file
3. This function sends a request to the translation server with the text to be translated and returns the translated text.
const translate = async (text) => {
const requestBody = {
'source': SOURCE_LANGUAGE,
'target': TARGET_LANGUAGE,
'q': text,
};
const options = {
'method': 'POST',
'headers': {'content-type': 'application/json'},
'body': JSON.stringify(requestBody),
};
try {
const response = await fetch(PRIVATE_TRANSLATOR_URL, options);
const responseJson = await response.json();
return responseJson['translatedText'];
} catch (error) {
throw new Error(`Translation failed: ${error.message}`);
}
};
4. This function recursively translates all strings in the JSON object. If the value is a string, the function translates it. If it's an array or object, it processes them accordingly.
const translateJsonDocument = async (value) => {
if (typeof value === 'string') {
return await translate(value);
}
if (value !== null && value !== undefined) {
let entries, valueNew;
if (Array.isArray(value)) {
entries = value.entries();
valueNew = [];
} else if (typeof value === 'object') {
entries = Object.entries(value);
valueNew = {};
}
if (entries !== undefined) {
for (const [key, item] of entries) {
valueNew[key] = await translateJsonDocument(item);
}
return valueNew;
}
}
return value;
};
5. This function reads the JSON file, parses and translates it using the previously defined functions, and prints the translated JSON to the console.
const main = async (jsonFilePath) => {
try {
const data = await fs.readFile(jsonFilePath, 'utf-8');
const jsonObject = JSON.parse(data);
const translated = await translateJsonDocument(jsonObject);
const translatedJson = JSON.stringify(translated, null, 4);
console.log(translatedJson);
} catch (error) {
console.error(`Error: ${error.message}`);
}
};
6. This line runs the main function with the specified path to the JSON file.
main(JSON_FILE_PATH);
Bash Implementation
For those who prefer working directly in the shell, here's a Bash script that accomplishes the same task.
But before you start, you will need the jq library to make working with JSON in BASH more convenient. This package is used in the code examples below. This is an example of how to install jq on Debian-based Linux distributions. You can install it using the following commands in the terminal on your machine.
sudo apt update
sudo apt install jq
1. These lines set the source language, target language, the private translation server's URL, and the JSON file's path.
SOURCE_LANGUAGE='en' # Source language
TARGET_LANGUAGE='fr' # Target language
PRIVATE_TRANSLATOR_URL='https://your-server-translator-url/api/translate' # Translation server URL
JSON_FILEPATH='/path-to-your-json-file' # Path to the JSON file
2. This function takes a text string as an argument and sends a POST request to the translation server. It uses curl to send the request and jq to parse the JSON response and extract the translated text. The function then echoes (returns) the translated text.
translate() {
local text="${1}"
local translated_text="$(curl -s -X POST "${PRIVATE_TRANSLATOR_URL}" \
-d "source=${SOURCE_LANGUAGE}" \
-d "target=${TARGET_LANGUAGE}" \
-d "q=${text}" | jq -r '.translatedText')"
echo "${translated_text}"
}
3. This function is the main part of the script:
- It checks if the JSON file exists. If not, it prints an error message and exits.
- It reads the JSON file into a variable.
- It extracts all scalar (string) values from the JSON using jq, storing them in variable lines.
- It iterates over each extracted entry, translates each value, and replaces the original value with the translated value in the JSON.
- Finally, it prints the modified JSON.
main() {
local json_file_path="${1}"
if [ ! -f "${json_file_path}" ]; then
echo "File not found: ${json_file_path}"
exit 1
fi
local json="$(cat "${json_file_path}")"
local lines="$(echo "${json}" | jq -c 'paths(scalars) as $p | getpath($p) as $v | select($v | type == "string") | {path: $p, value: $v}')"
while read entry; do
key="$(echo "${entry}" | jq -rc '.path')"
value="$(echo "${entry}" | jq -r '.value')"
translated_value="$(translate "${value}")"
json="$(echo "${json}" | jq --argjson key "${key}" --arg value "${translated_value}" 'setpath($key; $value)')"
done <<< "${lines}"
echo "${json}"
}
4. This line calls the main function with the path to the JSON file as an argument. It initiates the translation process for the JSON document.
main '${JSON_FILEPATH}'
Putting it to the Test: A Translation Example
Now that we've outlined the code for Python, JavaScript, and Bash, let's see a real example of this translation process in action. We'll use a sample JSON dataset representing product information and translate it from English to French.
Here's the input JSON data we'll be working with:
{
"products": [
{
"id": 1,
"name": "Wireless Mouse",
"description": "Ergonomic wireless mouse with adjustable DPI settings.",
"price": 25.99,
"category": "Electronics",
"stock": 150,
"reviews": [
{
"user": "JaneDoe",
"rating": 4.5,
"comment": "Great mouse, very responsive."
},
{
"user": "JohnSmith",
"rating": 4.0,
"comment": "Good value for the price."
}
]
},
{
"id": 2,
"name": "Mechanical Keyboard",
"description": "RGB backlit mechanical keyboard with customizable keys.",
"price": 79.99,
"category": "Electronics",
"stock": 85,
"reviews": [
{
"user": "AliceW",
"rating": 5.0,
"comment": "Fantastic typing experience!"
},
{
"user": "BobM",
"rating": 4.2,
"comment": "A bit noisy, but great quality."
}
]
}
]
}
We can feed this English JSON data into any of our code implementations (Python, JavaScript, or Bash). Each implementation uses the Lingvanex translation API to handle the language conversion.
After running the code, here's the translated JSON output we obtained:
{
"products": [
{
"id": 1,
"name": "Souris sans fil",
"description": "Souris sans fil ergonomique avec paramètres DPI réglables.",
"price": 25.99,
"category": "Electronique",
"stock": 150,
"reviews": [
{
"user": "JaneDoe",
"rating": 4.5,
"comment": "Excellente souris, très réactive."
},
{
"user": "JohnSmith",
"rating": 4.0,
"comment": "Bon rapport qualité-prix."
}
]
},
{
"id": 2,
"name": "Clavier mécanique",
"description": "Clavier mécanique rétroéclairé RVB avec touches personnalisables.",
"price": 79.99,
"category": "Electronique",
"stock": 85,
"reviews": [
{
"user": "AliceW",
"rating": 5.0,
"comment": "Expérience de frappe fantastique !"
},
{
"user": "BobM",
"rating": 4.2,
"comment": "Un peu bruyant, mais de grande qualité."
}
]
}
]
}
Key Points to Verify in the Output:
- Structure preservation: the overall organization and nesting of elements within the JSON should remain unchanged.
- Targeted translation: only the text-based fields intended for translation – such as product names, descriptions, and user reviews – should be in French. Numerical, boolean and other non-textual elements should remain in their original form.
- Accurate translation: ensure the translated French text accurately reflects the original English meaning.
By comparing the output JSON from each implementation and confirming these points, we can be sure our translation approach is robust, consistent, and ready for real-world use.
Important Considerations
As you proceed with your JSON translation, keep the following points in mind:
- Ensure that your private translation server URL is correct and accessible.
- Double-check the accuracy of your language codes to avoid translation errors.
- Verify that the file path to your JSON is correct to prevent script execution errors.
- Be aware that larger files may require more time for translation.
- It's advisable to have a human reviewer check the translated content for accuracy and context.
By following this guide, you've learned how to effectively translate JSON files using the Lingvanex On-premise Machine Translation Software. This method opens up numerous possibilities for localizing applications, translating datasets, and preparing content for global audiences.
Remember, while automated translation is powerful, it's always beneficial to have a human review of the final output. If you encounter any challenges or have questions about using the Lingvanex On-premise Machine Translation Software, don't hesitate to reach out to the Lingvanex support team for assistance.
You can see the scripts from the examples in full below:
- python_json_translator ›
import json
from collections.abc import Mapping, Sequence
import requests
_PRIVATE_TRANSLATOR_URL: str = 'https://your-server-translator-url/api/translate' # change to your translation server url
_SOURCE_LANGUAGE: str = 'en'
_TARGET_LANGUAGE: str = 'fr'
_JSON_FILEPATH: str = '/path-to-your-json-file' # change to your filepath to .json file
def _translate(text: str) -> str:
'''Translate text via private translator server'''
request_body = {
'source': _SOURCE_LANGUAGE,
'target': _TARGET_LANGUAGE,
'q': text,
}
with requests.post(url=_PRIVATE_TRANSLATOR_URL, data=request_body) as response:
response.raise_for_status() # In case of an error on the translator API side
return response.json()['translatedText']
def _translate_json_document(value: object) -> object:
'''
Recursively translates the object passed to this function.
If the passed object is Mapping - translates only the values.
:param value: One of the possible types that a JSON object can contain.
'''
if isinstance(value, str):
return _translate(value)
# Check if value is a List or a Dictionary and handle it appropriately
if isinstance(value, Mapping):
new_values = {}
iterable_values = value.items()
elif isinstance(value, Sequence):
new_values = [None]*len(value)
iterable_values = enumerate(value)
else:
return value
for key, item in iterable_values:
new_values[key] = _translate_json_document(item)
return new_values
def _main() -> None:
with open(_JSON_FILEPATH, 'r') as json_file:
data = json.load(json_file) # Convert JSON to Python object
translated = _translate_json_document(data)
translated_json = json.dumps(translated, indent=4, ensure_ascii=False)
print(translated_json)
# Uncomment code below in case if you need to write translated JSON to file
# target_translated_json_filepath: str = f'{_JSON_FILEPATH}.output.json' # Replace with your filepath
# with open(target_translated_json_filepath, 'w') as file:
# file.write(translated_json)
if __name__ == '__main__':
_main()
- javascript_json_translator ›
const fs = require('fs').promises
const SOURCE_LANGUAGE = 'en' // Set your source language here
const TARGET_LANGUAGE = 'fr' // Set your target language here
const PRIVATE_TRANSLATOR_URL = 'https://your-server-translator-url/api/translate' // Set your translator server URL here
const JSON_FILE_PATH = '/your-json-file-path' // change to your path to json file
const translate = async (text) => {
// Translate text via private translator server
const requestBody = {
'source': SOURCE_LANGUAGE,
'target': TARGET_LANGUAGE,
'q': text,
}
const options = {
'method': 'POST',
'headers': {'content-type': 'application/json'},
'body': JSON.stringify(requestBody),
}
try {
const response = await fetch(PRIVATE_TRANSLATOR_URL, options)
const responseJson = await response.json()
return responseJson['translatedText']
} catch(error) {
throw new Error(`Translation failed: ${error.message}`)
}
}
const translateJsonDocument = async (value) => {
// Recursively translates the object passed to this function.
// If the passed object is Mapping - translates only the values.
if (typeof value === 'string') {
return await translate(value)
}
// Check if value is an Array or a Dictionary and handle it appropriately
if (value !== null && value !== undefined) {
let entries, valueNew
if (Array.isArray(value)) {
entries = value.entries()
valueNew = []
} else if (typeof value === 'object') {
entries = Object.entries(value)
valueNew = {}
}
if (entries !== undefined) {
for (const [key, item] of entries) {
valueNew[key] = await translateJsonDocument(item)
}
return valueNew
}
}
return value
}
const main = async (jsonFilePath) => {
try {
const data = await fs.readFile(jsonFilePath, 'utf-8')
const jsonObject = JSON.parse(data)
const translated = await translateJsonDocument(jsonObject)
const translatedJson = JSON.stringify(translated, null, 4)
console.log(translatedJson)
} catch (error) {
console.error(`Error: ${error.message}`)
}
}
main(JSON_FILE_PATH)
- bash_json_translator ›
#!/usr/bin/env bash
SOURCE_LANGUAGE='en' # Set your source language here
TARGET_LANGUAGE='fr' # Set your target language here
PRIVATE_TRANSLATOR_URL='https://your-server-translator-url/api/translate' # Set your translator server URL here
JSON_FILEPATH='/path-to-your-json-file' # change to your filepath to .json file
translate() {
local text="${1}"
local translated_text="$(curl -s -X POST "${PRIVATE_TRANSLATOR_URL}" \
-d "source=${SOURCE_LANGUAGE}" \
-d "target=${TARGET_LANGUAGE}" \
-d "q=${text}" | jq -r '.translatedText')"
echo "${translated_text}"
}
main() {
local json_file_path="${1}"
if [ ! -f "${json_file_path}" ]; then
echo "File not found: ${json_file_path}"
exit 1
fi
local json="$(cat "${json_file_path}")"
local lines="$(echo "${json}" | jq -c 'paths(scalars) as $p | getpath($p) as $v | select($v | type == "string") | {path: $p, value: $v}')"
while read entry; do
key="$(echo "${entry}" | jq -rc '.path')"
value="$(echo "${entry}" | jq -r '.value')"
translated_value="$(translate "${value}")"
json="$(echo "${json}" | jq --argjson key "${key}" --arg value "${translated_value}" 'setpath($key; $value)')"
done <<< "${lines}"
echo "${json}"
}
main "${JSON_FILEPATH}"